How to Use Custom Colors Outside of Filament
If you want to use the blade components provided by the Filament package, they will not use the custom colors you defined in the Filament panel outside the Filament context by default. Here's how to do it:
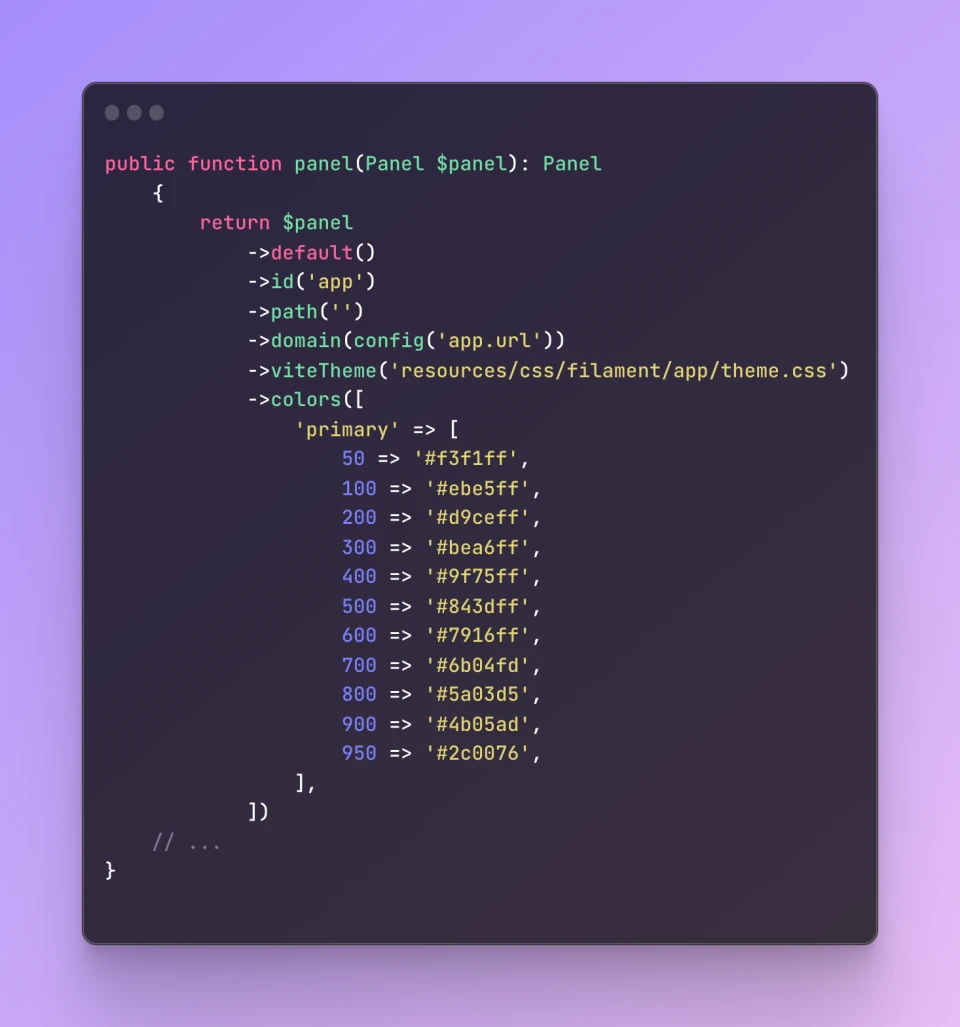
The Scenario
Let's say you defined the following custom primary color in your Filament (V3) AppPanelProvider.php:
1public function panel(Panel $panel): Panel 2 { 3 return $panel 4 ->default() 5 ->id('app') 6 ->path('') 7 ->domain(config('app.url')) 8 ->viteTheme('resources/css/filament/app/theme.css') 9 ->colors([10 'primary' => [11 50 => '#f3f1ff',12 100 => '#ebe5ff',13 200 => '#d9ceff',14 300 => '#bea6ff',15 400 => '#9f75ff',16 500 => '#843dff',17 600 => '#7916ff',18 700 => '#6b04fd',19 800 => '#5a03d5',20 900 => '#4b05ad',21 950 => '#2c0076',22 ],23 ])24 // ...25}
Now you want to use Filament components outside Filament, like described in the docs.
For example, a simple button:
1<x-filament::button2 href="https://filamentphp.com"3 tag="a">4 Filament5</x-filament::button>
The button will render with the default primary color of Filament (orange / yellow), because it's outside the Filament panel context.
How to solve it
In order to use still render your custom color, you need to define the colors inside a service provider, like so:
1use Filament\Facades\Filament; 2use Filament\Support\Facades\FilamentColor; 3 4 public function boot() 5 { 6 if (!Filament::isServing()) { 7 FilamentColor::register( 8 'primary' => [ 9 50 => '#f3f1ff',10 100 => '#ebe5ff',11 200 => '#d9ceff',12 300 => '#bea6ff',13 400 => '#9f75ff',14 500 => '#843dff',15 600 => '#7916ff',16 700 => '#6b04fd',17 800 => '#5a03d5',18 900 => '#4b05ad',19 950 => '#2c0076',20 ],21 );22 }23 }
This will ensure, that your custom colors are still used, when there's no Filament context.
Best Practice
Because I don't like to have duplicated code, I created a new config file filament-colors.php and placed my color definition there:
1<?php 2 3return [ 4 'primary' => [ 5 50 => '#f3f1ff', 6 100 => '#ebe5ff', 7 200 => '#d9ceff', 8 300 => '#bea6ff', 9 400 => '#9f75ff',10 500 => '#843dff',11 600 => '#7916ff',12 700 => '#6b04fd',13 800 => '#5a03d5',14 900 => '#4b05ad',15 950 => '#2c0076',16 ],17];
And used it in my AppPanelProvider.php and AppServiceProvider.php:
1public function panel(Panel $panel): Panel 2 { 3 return $panel 4 ->default() 5 ->id('app') 6 ->path('') 7 ->domain(config('app.url')) 8 ->viteTheme('resources/css/filament/app/theme.css') 9 ->colors(config('filament-colors'))10 // ...11}
1public function boot()2{3 if (!Filament::isServing()) {4 FilamentColor::register(5 config('filament-colors'),6 );7 }8}